4. MOVEMotor motor tests
Test defaults by not passing arguments. e.g.
buggy.forwards()
Test using named arguments. e.g.
buggy.forwards(speed=2, duration=200, decrease_left=5, decrease_right=0)
Test using named arguments not in order. e.g.
buggy.forwards(decrease_left=5, decrease_right=0, speed=2, duration=200)
Test using positional arguments. e.g
buggy.forwards(2, 200, 5, 0)
4.1. Set up the buggy
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
buggy.stop()
sleep(500)
4.2. forwards backwards test
- forwards(speed=1, duration=None, decrease_left=0, decrease_right=0)
- backwards(speed=1, duration=None, decrease_left=0, decrease_right=0)
for i in range(2, 11, 2)
to go forwards then backwards from speed 2 to speed 10 in steps of 2.from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def forward_backward_test():
# forwards(speed=1, duration=None, decrease_left=0, decrease_right=0)
# backwards(speed=1, duration=None, decrease_left=0, decrease_right=0)
for i in range(2, 11, 2):
buggy.forwards(i, 1000)
buggy.stop()
buggy.backwards(i, 1000)
buggy.stop()
buggy.stop()
sleep(2000)
forward_backward_test()
Tasks
Modify the code to just go forwards at increasing speeds.
Modify the code to just go backwards at increasing speeds.
Modify the code to go forwards at increasing speeds then backwards at increasing speeds.
4.3. Straight line test
decrease_left
when going forwards or backward:- forwards(speed=1, duration=None, decrease_left=0, decrease_right=0)
- backwards(speed=1, duration=None, decrease_left=0, decrease_right=0)
decrease_left
of 5 (delta) and vary this manually until a straight line is achieved.from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def straight_line_test(delta=5):
# straight line test with smooth start and stop
buggy.forwards(speed=2, duration=200, decrease_left=delta, decrease_right=0)
buggy.forwards(5, 200, delta, 0)
buggy.forwards(9, 1000, delta, 0)
buggy.forwards(5, 200, delta, 0)
buggy.forwards(2, 200, delta, 0)
buggy.stop()
buggy.backwards(2, 200, delta, 0)
buggy.backwards(5, 200, delta, 0)
buggy.backwards(9, 1000, delta, 0)
buggy.backwards(5, 200, delta, 0)
buggy.backwards(2, 200, delta, 0)
buggy.stop()
sleep(2000)
straight_line_test(5)
Tasks
Modify the code to use
decrease_right
instead ofdecrease_left
.Modify the code to use a for-loop to test delta values of 5, 10, 15, and 20.
4.4. Individual motors test
- left_motor(speed=1, duration=None)
- right_motor(speed=1, duration=None)
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def individual_motors_test():
# left_motor(speed=1, duration=None)
# right_motor(speed=1, duration=None)
for i in range(-10, 11, 2):
buggy.left_motor(i, 200)
buggy.stop()
for i in range(10, -11, -2):
buggy.right_motor(i, 200)
buggy.stop()
sleep(2000)
individual_motors_test()
Tasks
Modify the code to just use the left motor.
Modify the code to just use the right motor.
Modify the for-loops for each motor to just go forwards at varying speeds.
Modify the for-loop for each motor to just go backwards at varying speeds.
Modify the for-loop to change the speed in steps of 1 for 100ms each.
Modify the for-loop to change the speed in steps of 5 for 400ms each.
4.5. Spin test
- spin(speed=1, duration=None)
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def spin_test():
# spin(speed=1, duration=None)
for i in range(2, 11, 2):
buggy.spin_left(i, 500)
buggy.stop()
for i in range(2, 11, 2):
buggy.spin_right(i, 500)
buggy.stop()
sleep(2000)
spin_test()
Tasks
Modify the code to just spin left.
Modify the code to just spin right.
Modify the code to spin left then right at each speed as the speed of spinning is increased.
4.6. Turn test
- left(speed=1, radius=25, duration=None)
- right(speed=1, radius=25, duration=None)
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def turn_test():
# left(speed=1, radius=25, duration=None)
# right(speed=1, radius=25, duration=None)
for i in range(2, 11, 2):
buggy.left(i, 25, 400)
buggy.stop()
for i in range(2, 11, 2):
buggy.right(i, 25, 400)
buggy.stop()
sleep(2000)
turn_test()
Tasks
Modify the code to just turn left.
Modify the code to just turn right.
Modify the code to turn left then right at each speed as the speed of turning is increased..
4.7. Zig Zag test
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def zigzag_test(slow_speed=2, fast_speed=4, zigzag_count=5, zigzag_time=1000):
for i in range(zigzag_count):
buggy.left_motor(fast_speed)
buggy.right_motor(slow_speed)
sleep(zigzag_time)
buggy.left_motor(slow_speed)
buggy.right_motor(fast_speed)
sleep(zigzag_time)
for i in range(zigzag_count):
buggy.left_motor(-slow_speed)
buggy.right_motor(-fast_speed)
sleep(zigzag_time)
buggy.left_motor(-fast_speed)
buggy.right_motor(-slow_speed)
sleep(zigzag_time)
buggy.stop()
sleep(2000)
zigzag_test(2, 4, 5, 1000)
Tasks
Modify the code to just zig zag forward.
Modify the new zig zag forwards code with
zigzag_count=1
, and placezigzag_test(2, fast_speed, 5, 1000)
in a for-loop and varyfast_speed
from 3 to 8.
4.8. Polygon test
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def polygon_test(spin_duration=240, sides=20):
for i in range(sides):
buggy.forwards(3, 800)
buggy.spin(1, 'left', spin_duration)
buggy.stop()
sleep(2000)
polygon_test(240)
Tasks
Experiment with the spin duration value to move the buggy in the shape of a square.
Experiment with the spin duration value to move the buggy in the shape of a triangle.
4.9. Spiral test
from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def spiral_test():
for i in [10, 20, 40, 60, 80, 100]:
buggy.left(5, i, duration=1000)
buggy.stop()
sleep(2000))
spiral_test()
Tasks
Edit the radii values in the list to create different spirals.
Edit the radii values in the list to spiral inwards.
4.10. Oval test
radii
and a list of durations
so that both lists can be used in a for-loop.for r, d in zip(radii, durations)
to create an iterator that produces tuples of the form (r, d)
from the two lists: radii
and durations
.radii
has values for each expected radius for the turn.durations
has values for each expected duration for the turn.from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def oval_test():
radii = [20, 40, 60, 80, 60, 40]
durations = [500, 600, 1000, 1000, 1000, 600]
for i in range(6):
for r, d in zip(radii, durations):
buggy.left(3, r, d)
buggy.stop()
sleep(2000)
oval_test()
Tasks
Experiment with the radii or durations to vary the oval shape.
4.11. Loops test
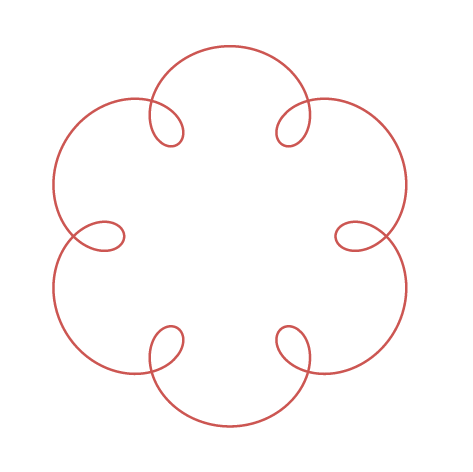
for r, d in zip(radii, durations)
to create an iterator that produces tuples of the form (r, d)
from the two lists: radii and durations.radii
has values in order for each expected radius for the turn.durations
has values in order for each expected duration for the turn.from microbit import *
import MOVEMotor
# setup buggy
buggy = MOVEMotor.MOVEMotorMotors()
def loops_test():
radii = [10, 30, 80, 30]
durations = [2000, 400, 1200, 400]
for i in range(6):
for r, d in zip(radii, durations):
buggy.left(5, r, d)
buggy.stop()
sleep(2000)
loops_test()
Tasks
Experiment with the radii or durations to vary the epitrochoid’s shape.